Note
Click here to download the full example code
Getting started: 5 minutes tutorial for tofu¶
This is a tutorial that aims to get a new user a little familiar with tofu’s structure.
# The following imports matplotlib, preferably using a
# backend that allows the plots to be interactive (Qt5Agg).
import numpy as np
We start by loading tofu. You might see some warnings at this stage since optional modules for tofu could be missing on the machine you are working on. This can be ignored safely.
import tofu as tf
We can now create our first configuration. In tofu speak, a configuration is the geometry of the device and its structures. tofu provides pre-defined ones for your to try, so we’re going to do just that:
configB2 = tf.load_config("B2")
The configuration can easily be visualized using the .plot() method:
configB2.plot()
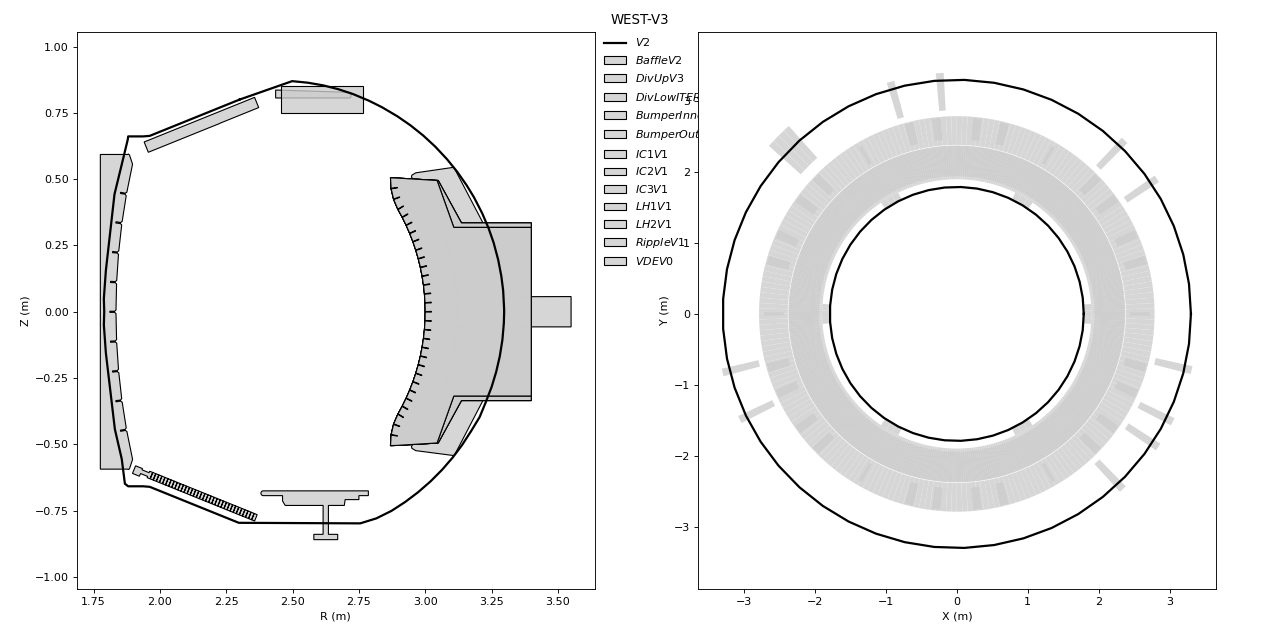
Out:
/home/lasofivec/tofu/tofu/geom/_def.py:127: MatplotlibDeprecationWarning:
The set_window_title function was deprecated in Matplotlib 3.4 and will be removed two minor releases later. Use manager.set_window_title or GUI-specific methods instead.
f.canvas.set_window_title(wintit)
[<AxesSubplot:xlabel='R (m)', ylabel='Z (m)'>, <AxesSubplot:xlabel='X (m)', ylabel='Y (m)'>]
Since tofu is all about tomography, let’s create a 1D camera and plot its output.
cam1d = tf.geom.utils.create_CamLOS1D(
config=configB2,
pinhole=[3.4, 0, 0],
sensor_nb=100,
focal=0.1,
sensor_size=0.1,
orientation=[np.pi, 0, 0],
Name="",
Exp="",
Diag="",
)
# interactive plot
cam1d.plot_touch()
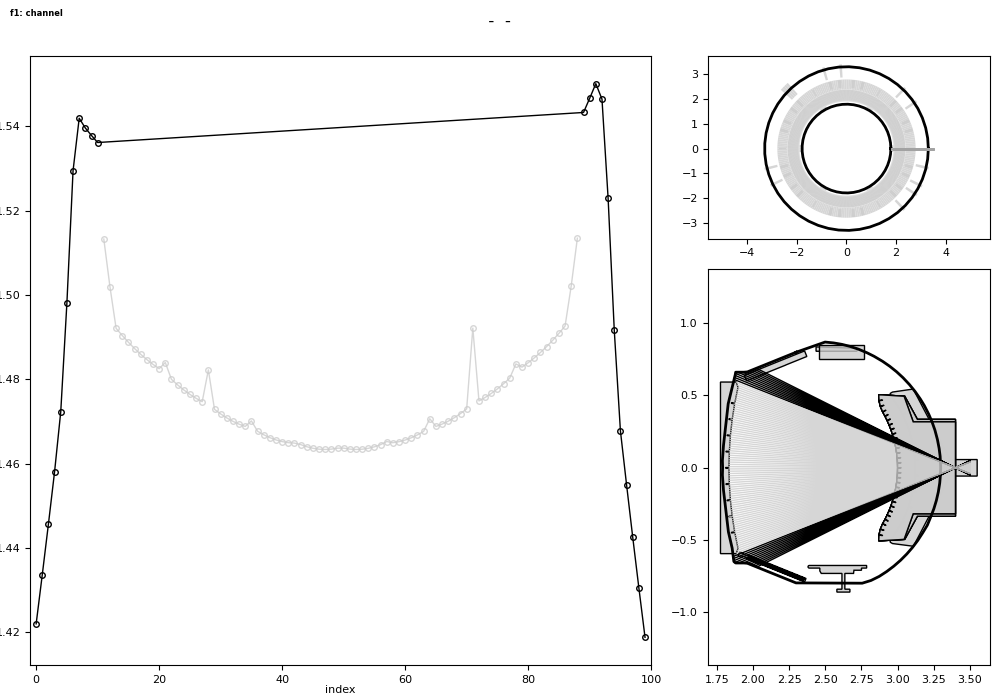
Out:
/home/lasofivec/miniconda3/envs/newtofu3/lib/python3.9/site-packages/numpy/core/fromnumeric.py:3208: VisibleDeprecationWarning: Creating an ndarray from ragged nested sequences (which is a list-or-tuple of lists-or-tuples-or ndarrays with different lengths or shapes) is deprecated. If you meant to do this, you must specify 'dtype=object' when creating the ndarray.
return asarray(a).size
/home/lasofivec/tofu/tofu/geom/_plot.py:1412: MatplotlibDeprecationWarning:
The set_window_title function was deprecated in Matplotlib 3.4 and will be removed two minor releases later. Use manager.set_window_title or GUI-specific methods instead.
fig.canvas.set_window_title(wintit)
/home/lasofivec/tofu/tofu/utils.py:3476: UserWarning: Not interactive backend!:
- backend : agg (prefer Qt5Agg)
- canvas : FigureCanvasAgg
warnings.warn(msg)
<tofu.utils.KeyHandler_mpl object at 0x7f31585b4790>
The principle is similar for 2D cameras.
cam2d = tf.geom.utils.create_CamLOS2D(
config=configB2,
pinhole=[3.4, 0, 0],
sensor_nb=100,
focal=0.1,
sensor_size=0.1,
orientation=[np.pi, 0, 0],
Name="",
Exp="",
Diag="",
)
cam2d.plot_touch()
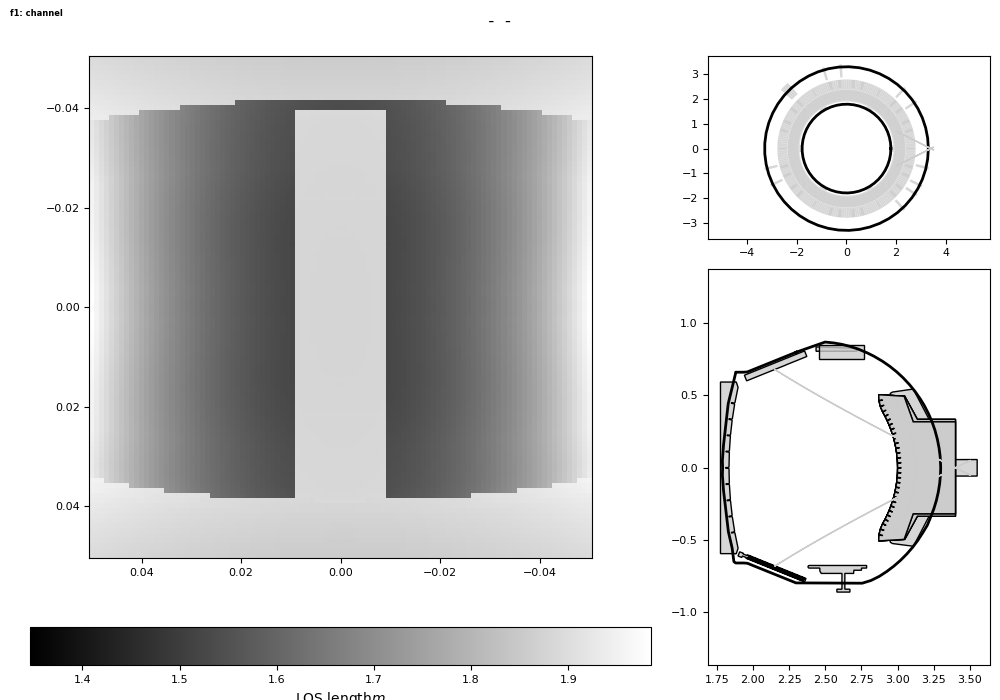
Out:
/home/lasofivec/miniconda3/envs/newtofu3/lib/python3.9/site-packages/numpy/core/fromnumeric.py:3208: VisibleDeprecationWarning: Creating an ndarray from ragged nested sequences (which is a list-or-tuple of lists-or-tuples-or ndarrays with different lengths or shapes) is deprecated. If you meant to do this, you must specify 'dtype=object' when creating the ndarray.
return asarray(a).size
/home/lasofivec/tofu/tofu/geom/_plot.py:1412: MatplotlibDeprecationWarning:
The set_window_title function was deprecated in Matplotlib 3.4 and will be removed two minor releases later. Use manager.set_window_title or GUI-specific methods instead.
fig.canvas.set_window_title(wintit)
/home/lasofivec/tofu/tofu/utils.py:3476: UserWarning: Not interactive backend!:
- backend : agg (prefer Qt5Agg)
- canvas : FigureCanvasAgg
warnings.warn(msg)
<tofu.utils.KeyHandler_mpl object at 0x7f31685bc670>
What comes next is up to you! You could now play with the function parameters (change the cameras direction, refinement, aperture), with the plots (many are interactive) or create you own tomographic configuration.
Total running time of the script: ( 0 minutes 6.412 seconds)